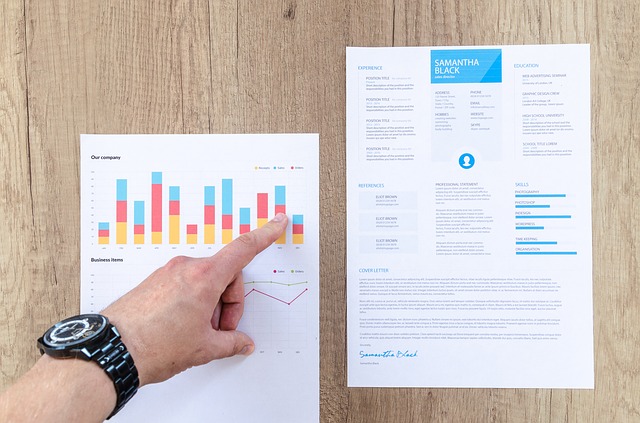
In order to Get all sites from within an SharePoint Online tenancy we can run a pretty simple PowerShell script which then exports all of the information into a text file.
This is great for auditing purposes:
[/csharp]
#Required Parameters
$sUserName = “username@domain.com”
$sPassword = Read-Host -Prompt “Enter your password: ” -AsSecureString
$sSiteUrl = “https://tenant-admin.sharepoint.com/”
$host.Runspace.ThreadOptions = “ReuseThread”
# Set Transcript file name
$Now = Get-date -UFormat %Y%m%d_%H%M%S
$File = “c:\PublishFilesSPO_$Now.txt”
#Start Transcript
Start-Transcript -path $File | out-null
#Definition of the function that gets the list of site collections in the tenant using CSOM
function Get-SPOTenantSiteCollections
{
param ($sSiteUrl,$sUserName,$sPassword)
try
{
Write-Host “—————————————————————————-” -foregroundcolor Green
Write-Host “Getting the Tenant Site Collections” -foregroundcolor Green
Write-Host “—————————————————————————-” -foregroundcolor Green
#Adding the Client OM Assemblies
Add-Type -Path “c:\Program Files\Common Files\microsoft shared\Web Server Extensions\15\ISAPI\Microsoft.SharePoint.Client.dll”
Add-Type -Path “c:\Program Files\Common Files\microsoft shared\Web Server Extensions\15\ISAPI\Microsoft.SharePoint.Client.Runtime.dll”
Add-Type -Path “c:\Program Files\Common Files\microsoft shared\Web Server Extensions\15\ISAPI\Microsoft.Online.SharePoint.Client.Tenant.dll”
#SPO Client Object Model Context
$spoCtx = New-Object Microsoft.SharePoint.Client.ClientContext($sSiteUrl)
$spoCredentials = New-Object Microsoft.SharePoint.Client.SharePointOnlineCredentials($sUsername, $sPassword)
$spoCtx.Credentials = $spoCredentials
$spoTenant= New-Object Microsoft.Online.SharePoint.TenantAdministration.Tenant($spoCtx)
$spoTenantSiteCollections=$spoTenant.GetSiteProperties(0,$true)
$spoCtx.Load($spoTenantSiteCollections)
$spoCtx.ExecuteQuery()
#We need to iterate through the $spoTenantSiteCollections object to get the information of each individual Site Collection
foreach($spoSiteCollection in $spoTenantSiteCollections){
if ($spoSiteCollection.Url -like “*teams*” -or $spoSiteCollection.Url -like “*sites*”){
Write-Host “Url: ” $spoSiteCollection.Url ” – Template: ” $spoSiteCollection.Template ” – Owner: ” $spoSiteCollection.Owner
}
}
$spoCtx.Dispose()
}
catch [System.Exception]
{
write-host -f red $_.Exception.ToString()
}
}
Get-SPOTenantSiteCollections -sSiteUrl $sSiteUrl -sUserName $sUserName -sPassword $sPassword
[/csharp]