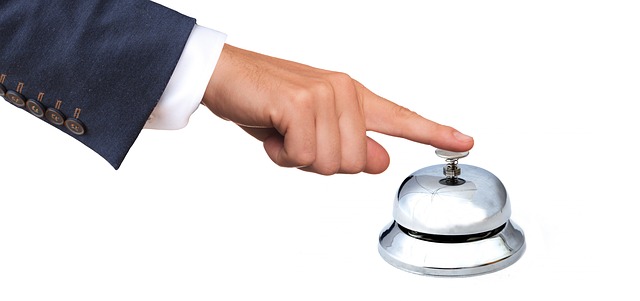
Use the code below to programmatically check-in and publish all files within a document library in SharePoint Online
[csharp]</p> <p># Clear the screen<br> Clear-Host</p> <p># Add Wave16 references to SharePoint client assemblies – required for CSOM<br> Add-Type -Path "c:\Program Files\Common Files\microsoft shared\Web Server Extensions\15\ISAPI\Microsoft.SharePoint.Client.dll"<br> Add-Type -Path "c:\Program Files\Common Files\microsoft shared\Web Server Extensions\15\ISAPI\Microsoft.SharePoint.Client.Runtime.dll" </p> <p># Parameters<br> # Specify the subsite URL where the list/library resides<br> $SiteUrl = Read-Host -Prompt "URL in format of https://tenant.sharepoint.com/"<br> # Title of the List/Library you want to publish all files within<br> $ListName = "Master Page Gallery"</p> <p># Username with sufficient publish/approve permissions<br> $UserName = "username@mydomain.com"<br> # User will be prompted for password</p> <p># Set Transcript log file name<br> $Now = Get-date -UFormat %Y%m%d_%H%M%S<br> #choose where to save the file and the name it will have ($Now is a variable in the field name below)<br> $File = "c:\PublishFilesSPO_$Now.txt"<br> #Start Transcript<br> Start-Transcript -path $File | out-null</p> <p># Display the data to the user<br> Write-Host "/// Values entered for use in script ///" -foregroundcolor cyan<br> Write-Host "Site: " -foregroundcolor white -nonewline; Write-Host $SiteUrl -foregroundcolor green<br> Write-Host "List name: " -foregroundcolor white -nonewline; Write-Host $ListName -foregroundcolor green<br> Write-Host "Useraccount: " -foregroundcolor white -nonewline; Write-Host $UserName -foregroundcolor green<br> # Prompt User for Password<br> $SecurePassword = Read-Host -Prompt "Password" -AsSecureString<br> Write-Host "All files in " -foregroundcolor white -nonewline; Write-Host $ListName -foregroundcolor green -nonewline; Write-Host " on site " -foregroundcolor white -nonewline; Write-Host $SiteUrl -foregroundcolor green -nonewline; Write-Host " will be published by UserName " -foregroundcolor white -nonewline; Write-Host $UserName -foregroundcolor green<br> Write-Host " "</p> <p># Prompt to confirm<br> Write-Host "Are these values correct? (Y/N) " -foregroundcolor yellow -nonewline; $confirmation = Read-Host</p> <p># Run script when user confirms<br> if ($confirmation -eq ‘y’) {</p> <p> # Bind to site collection<br> $Context = New-Object Microsoft.SharePoint.Client.ClientContext($SiteUrl)<br> $credentials = New-Object Microsoft.SharePoint.Client.SharePointOnlineCredentials($UserName, $SecurePassword)<br> $Context.Credentials = $credentials</p> <p> # Bind to list<br> $list = $Context.Web.Lists.GetByTitle($ListName)<br> # Query for All Items<br> $query = New-Object Microsoft.SharePoint.Client.CamlQuery<br> $query.ViewXml = " "<br> $collListItem = $list.GetItems([Microsoft.SharePoint.Client.CamlQuery]::CreateAllItemsQuery())<br> $Context.Load($List)<br> $Context.Load($collListItem)<br> $Context.ExecuteQuery()</p> <p> # Go through process for all items<br> foreach ($ListItem in $collListItem){<br> # Adding spacer<br> Write-Host " "<br> Write-Host "/////////////////////////////////////////////////////////////"<br> Write-Host " "<br> # Write the Item ID, the FileName and the Modified date for each items which is will be published<br> Write-Host "Working on file: " -foregroundcolor yellow -nonewline; Write-Host $ListItem.Id, $ListItem["FileLeafRef"], $ListItem["Modified"], $ListItem["FileRef"]</p> <p> # Un-comment below if you want to add a filter to skip all files with a certain phrase in the name<br> # if ($ListItem["FileRef"] -notlike "*Team_MWS*"){<br> # Write-Host "Skip file" -foregroundcolor red<br> # continue<br> # }<br> #Write-Host $ListItem | Get-Member<br> #<br> # Un-comment below if you want to add a filter to skip all files which where modifed last < 31-jan-2015<br> # $ListItem["Modified"] -lt "01/31/2015 00:00:00 AM"){<br> # Write-Host "This item was last modified before January 31st 2015" -foregroundcolor red<br> # Write-Host "Skip file" -foregroundcolor red<br> # continue<br> # }</p> <p> # Check if file is checked out by checking if the "CheckedOut By" column does not equal empty</p> <p> if ($ListItem["CheckoutUser"] -ne $null){<br> #$item = $ListItem.ListItemAllFields<br> #Write-Host $item["Title"]<br> Write-Host $ListItem["FileLeafRef"]<br> Write-Host "File is checked Out"</p> <p> # Item is not checked out, Check in process is applied<br> Write-Host "File: " $ListItem["FileLeafRef"] "is checked out." -ForegroundColor Cyan<br> $listItem.File.CheckIn("Auto check-in by PowerShell script", [Microsoft.SharePoint.Client.CheckinType]::MajorCheckIn)<br> Write-Host "- File Checked in" -ForegroundColor Green<br> }<br> if ($ListItem["MinorVersion"] -ne $null){<br> # Publishing the file<br> Write-Host "Publishing file:" $ListItem["FileLeafRef"] -ForegroundColor Cyan<br> $listItem.File.Publish("Auto publish by PowerShell script")<br> Write-Host "- File Published" -ForegroundColor Green<br> }</p> <p> # Check if the file is approved by checking if the "Approval status" column does not equal "0" (= Approved)<br> if ($List.EnableModeration -eq $true){<br> # if Content Approval is enabled, the file will be approved<br> if ($ListItem["_ModerationStatus"] -ne ‘0’){<br> # File is not approved, approval process is applied<br> Write-Host "File:" $ListItem["FileLeafRef"] "needs approval" -ForegroundColor Cyan<br> $listItem.File.Approve("Auto approval by PowerShell script")<br> Write-Host "- File Approved" -ForegroundColor Green<br> }<br> else {<br> Write-Host "- File has already been Approved" -ForegroundColor Green<br> }<br> }<br> if ($listItem) {<br> $Context.Load($listItem)<br> $Context.ExecuteQuery()<br> } else { ‘Write-Host "- File already published" -ForegroundColor Green’ }</p> <p> }<br> # Adding footer<br> Write-Host " "<br> Write-Host "/////////////////////////////////////////////////////////////"<br> Write-Host " "<br> Write-Host "Script is done" -ForegroundColor Green<br> Write-Host "Files have been published/approved" -ForegroundColor Green<br> }<br> # Stop script when user doesn’t confirm<br> else {<br> Write-Host " "<br> Write-Host "Script cancelled by user" -foregroundcolor red<br> Write-Host " "<br> }<br> Stop-Transcript | out-null<br> [/csharp]